My First Coding Adventure
So, I said I was going to make a game...
Up to this point, I’ve been working on trying to figure out what that game is. I’m pretty confident that I’ve figured that out now, and so I needed to actually start making it.
To recap, in the game (let’s stick with the working title: BOXBOT) you play as a robot, made out of a cardboard box by an obsessive box-collector, who ventures out into the world to collect boxes for their creator. Each level is a puzzle where, when you collect each goal box, it is stacked onto your head, changing your size, shape and weight depending on the order that you pick up the boxes. This will mean you need to collect the boxes in the right order in order to exit the level with the boxes.
With that slightly convoluted elevator pitch put together, I decided it was time to start programming a prototype of the game. This is the beginning of my first coding adventure.
There’s a really great YouTuber called Sebastian Lague, who has a series of videos he calls Coding Adventures. In each video, rather than creating a tutorial explaining how he programs something, he takes you on a journey through his process of figuring out how to program something – or sometimes even just exploring an idea through coding. They’ve been a really great resource for me – not because I’m about to start writing my own procedural erosion program for simulating water eroding mountains as a way of generating an environment – but because he really demonstrates what is, in my eyes, a more realistic approach to a coding workflow.
https://youtu.be/lctXaT9pxA0
As someone who has literally never programmed something on their own before, most tutorials don’t really explain how to figure things out. All the introductions to C# (Unity's programming language) that I’ve worked through pretty much tell you exactly what to write. It’s a useful way to be introduced to the tools of the programming language, and to start to wrap your head around its syntax, but when you sit down with a unique goal you came up with yourself, you can find yourself a little bit lost for how to begin.
That’s where the “coding adventure” approach comes into play. Rather than trying to simply write the code I needed to make my game, I instead decided to approach this problem as trying to figure out what code I could use to try to make a prototype of my idea work. It might sound like the same thing, but by framing the goal differently in my mind, I was able to get over feeling “stumped” about how to start and just dive in. My goal wasn’t to make it work, my goal was to start figuring out how to make it work.
And so let me take you through my process of trying to set up the most basic functionality I needed for my game: moving the character and picking up boxes.
Coding Adventure: Picking up a cube
For now, I’m using some extremely placeholder elements. Everything is just plain boxes which will later be replaced with actual characters and props (more on that later).
Character movement is something I had enough experience with from tutorials that I knew how to get started with it. I wrote a little script that uses Unity’s physics engine to apply a force to the player when you press the left or right arrows on the keyboard. This results in the “player” (box) moving left and right based on your input! I’m not super happy with the feel of the movement at this point, but it works and I can figure out how to fine tune that later on.
Here’s a snippet of what that movement code looks like:
public class PlayerController : MonoBehaviour
{
private Rigidbody playerRb;
public float movementSpeed = 5.0f;
void Start()
{
playerRb = GetComponent<Rigidbody>();
}
void Update()
{
// MOVEMENT: I need to create a force in the horizontal axis when the player hits the corresponding buttons (left/right)
// this is a variable that accesses the horizontal axis
float horizontalInput = Input.GetAxis("Horizontal");
// this is how I add the force, multiplied by the speed and direction, to the character. Not sure if I want Vector3.forward or something else. I also have no idea if Acceleration is actually the right ForceMode, but I can experiment with that later
playerRb.AddForce(Vector3.right * movementSpeed * horizontalInput, ForceMode.Acceleration);
}
}
Now that my “player” could move around, I needed to add boxes that they could collect. The key thing here was that I wanted the box to be picked up based on a button input (so it isn’t picked up automatically), and rather than being put into an inventory and disappearing, the box would be moved to a position above the player and stacked on their head. Sounds simple, right? I’m sure to someone with any experience it is, but this was the days long process for how I figured out something that “works”. For now. Kind of.
To start with, I knew I needed to have some kind of way of knowing what box I was close to and could pick up. I’ve seen people use a technique for different things, so I tried creating something called a "trigger". Basically, I attached a spherical collider in a radius around the box and made it a “trigger”, which means I can use my player’s collider to detect when I’ve entered this spherical boundary. When the player enters a pickup’s “pickup radius”, the game sets that box as an object that the player can pick up. This kind of weird circular logic is something that I’m starting to get used to the more that I work on coding, but it isn’t super intuitive to me still. It’s weird to think about needing to tell the computer that an object is in range of my player when you kind of take that stuff for granted in a finished game. Of course it’s in range, I can see it right there!
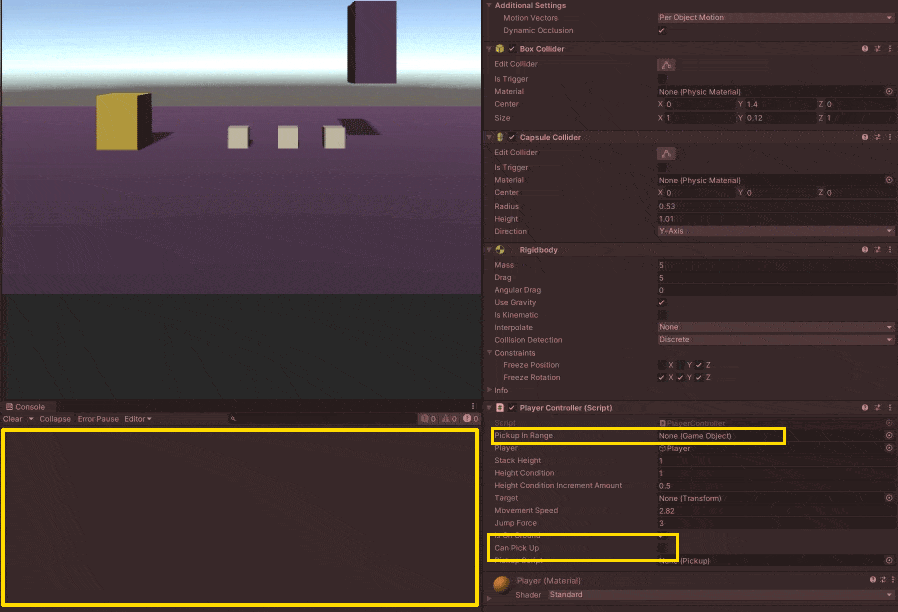
Once my player is in range of the pickup, I created a condition where, if they press the “pickup” button, the box that has been marked as “can be picked up” will get picked up. The only thing is, there isn’t a built-in method in C# for just picking something up, so I had to figure out how to do that, too.
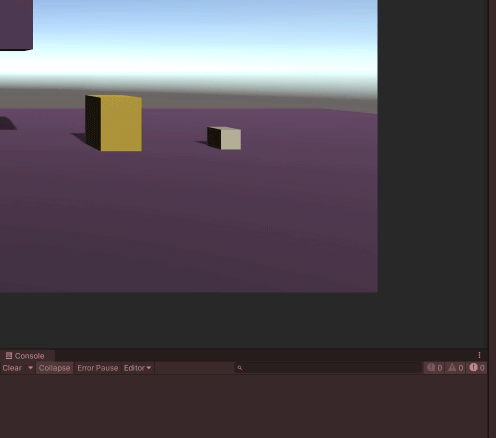
This is where googling comes into play. Actually, googling has always been in play. I’m not sure if any of this would be possible without googling, and it is the most valuable skill I’ve learned from my years of professional design and animation work – never be too proud to google how to do something. Accessing the infinite resource of the internet to figure things out is like a super power that we all have, and it isn’t even a default skill that we’re all good at. Learning how to google for helpful information is actually a really valuable skill, too.
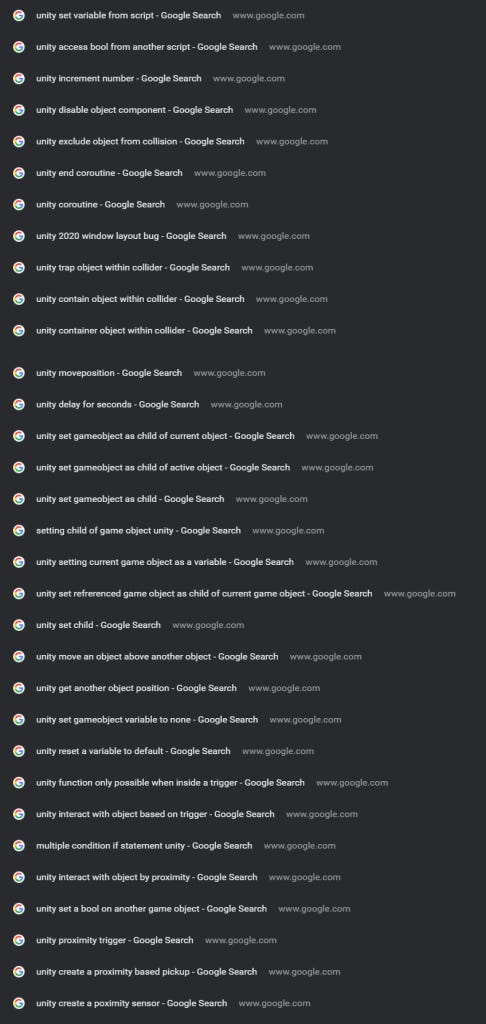
All this work and we still haven’t even tried to pick up the box yet! From this point, I experimented with a few different methods for picking up this stupid box and had a variety of fun broken results.
https://videopress.com/v/WOv2PVop?preloadContent=metadata
Eventually, I actually managed to arrive at something that sort of works. It at least delivers the expected result (with one known bug where, if you are too close to the pick up it will attach to the player before it gets moved to their head) and I was even able to get this to work for multiple boxes!
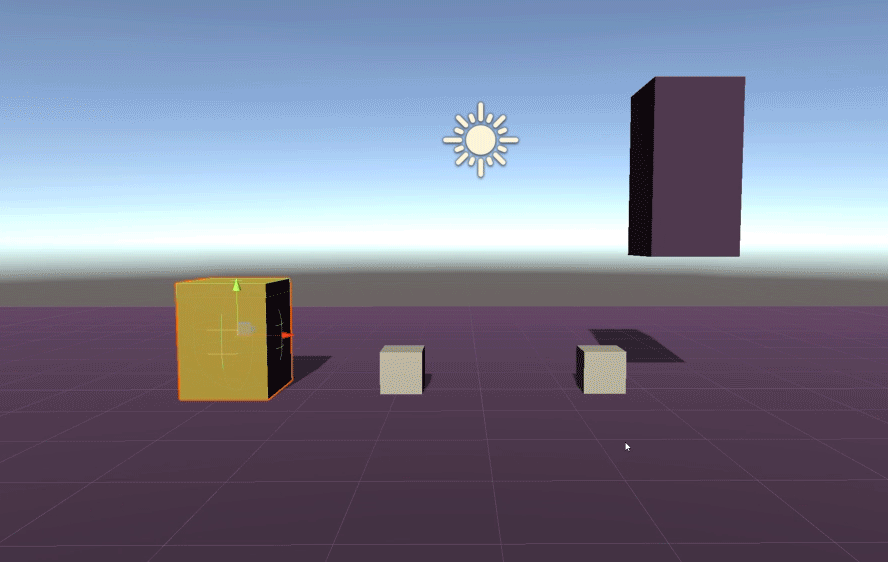
And that’s my first major coding adventure! At this point, I’m feeling really pleased and also very sure that there are better ways to make this happen. One thing I’m not sure about is whether or not I want these boxes to remain as physics objects or not. I think the only way to figure that out is to push forward and rework things if I start to run into issues with the way I’ve put this together.
I'm already thinking of multiple different ways I could achieve the same result here and maybe avoid some of the issues I've been running into, and part of the fun of this entire process is how open-ended everything is! I don't think you could really say there is a right way to do anything – though I'm sure everyone believes they know the right way...
At this point I decided to take a pause from the programming to start working on some art. More on that next time!